The fad behind the Arrays and the generic Collections is defined by this two important scenarios: Arrays are covariant(Widening their reference) while Collections are invariants(Narrowing their reference). When it comes to input parameters it becomes clear that these two containers differ largely and requires extra careful approach. for instance if a class of Foo is a subtype of another class Foobar then the array of type Foo[] is a subtype of Foobar[].
Dec 4, 2012
Nov 8, 2012
Java 7 Overview: Multi-Catch Support
Java 7, also known as Project Coin also improved Java's exception handling with the introduction of Muti-Catch Exception and Final Rethrow supports. With Multi-catch you will now be able to catch multiple exceptions in single block contrary to Java 6 or earlier where this isn't possible.
In the previous posts, I used a multicatch feature in the Cryptography examples.
Despite the fact that in the previous code, the method encrypt_data() throws an exception.
Nov 6, 2012
Java 7 Overview: Automatic Resource Management
Java 7 support Automatic Resources Management through the try-with-resources statement. A resource is an object that must be closed after the program is finished with it. The try statement could declare one or more resources, where it ensures that each resource is closed at the end of the statement. Any object that implements java.lang.AutoCloseable, which includes all objects which implement java.io.Closeable, can be used as a resource in this regard.
Nov 2, 2012
Java 7 Overview: String in Switch Support
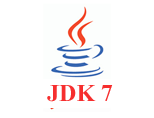
The issue worth mentioning about switch statement when used with a String type is, it uses equals() method to compare the expression pass to it, with each value in the case statement, which made it case-sensitive and will throw a NullPointerException if the expression is null.
As a result, checking for null parameter and converting the string to lower
Oct 9, 2012
Simple JMF Media Player
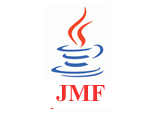
Oct 5, 2012
Data Encryption in Java Part 2
This is another Data Encryption example in Java, but it featured DES encryption algorithm which is academically popular and has been in use for quite some time. DES uses 56-bits key size and also uses a key to customize the transformation, so that decryption can only be performed by those who know the particular key used for the encryption. Every 8th bit of the selected key is discarded, that is, positions 8, 16, 24, 32, 40, 48, 56, 64 are removed from the 64 bit key leaving behind only the 56 bit key.
Oct 3, 2012
How to Create System Tray Icon in Java
In this tutorial, I will show you how to create a System Tray Icon in Java. Operating Systems normally provides a place(program) for icons display, either for the programs that runs in background, such as services, or programs that continue to run while the window is closed. For example, if your computer supports advanced power management (APM),
a Battery Meter icon may be displayed on the taskbar. In Windows its called a Status Area, others called it System Tray.
Sep 29, 2012
Data Encryption in Java Part 1
In this example, I will show you how to encrypt data in Java using Blowfish Cipher algorithm.
Blowfish is a keyed, symmetric block cipher, with 64-bit block sized encryption algorithm, with a variable key length from 32 bits up to 448 bits. Blowfish provides a good encryption rate in software.
It is meant to be an alternative to DES(Data Encryption Standard) which is the predominantly used algorithm for the data encryption developed at IBM in the early 1970s.
Sep 27, 2012
How to Create your own Splash Screen in Java
In this program, I will show you how to create your own Non-SDK Splash Screen. If you found the Java Splash Screen APIs hard to implement, this is a good choice for you.
Splash Screen is the short lived window that pops out in the middle of the computer or mobile device before the main program is fully loaded and lunched to the user. It mostly contains the progress of the loading components built in the program or just a logo of the software.
Sep 24, 2012
Auto-Scrolling Texts with Image Background in Java
This is an example of how to program an animated texts, that automatically
scrolls up slowly with an image as its background. In this Java program I used
threading, the IO and math APIs to bring everything together. First, the texts are
loaded into the stream, pull every line of it and then draw it on to the screen.
A top and bottom offset is used to nicely cut the starting as well as the ending
points of the texts, as they scrolls in a given time interval.
Sep 15, 2012
How To Load And Display Image From File In Java
In this Java tutorial, we set to graphically display image from a directory. We first set a file reference to our image directory, in this case we gonna use BufferedImage object to get the decoded buffered image, automatically invoking ImageReader.
You can see no ImageInputStream is physically used, this is because the file is wraped in an image input stream as soon as it register the file to the ImageReader object. Null is returned if the registration failed.
Sep 13, 2012
How To Make a Copy of File in Java
In this simple example, a file is loaded into a stream, copy it into a temporary storage called "temp", from which it was emptied bit by bit to a new directory.
Sep 4, 2012
Smart Calculator
Calculator is one of the applications that every programmer want to create, it is fun to see how a simple code can make such an awesome arithmetic operations. People write calculator code in different ways, depending on the kind of language you are familiar with, and how easy or otherwise that language can avail you that, as well as how complex the operation would be. Java has a great library for UX and maths, which is great for developing calculator or other educational business/ applications.
Sep 2, 2012
Camera Application Using JMF
This a simple Java program that opens the system
Webcam and presents the visual data of the camera. It used Java Media
Framework library, though has not been updated for a very long, but
developers still found it very useful for capture,
playback, stream, and transcoding multiple media formats. You need to
integrate the JMF plugin into your project in order to use the JMF APIs.
You can download the JMF library here at Softpedia.
May 18, 2012
Accessing Private Member From Other Class In Java
Many people wonder if a private field can be accessed from other completely strange class. The words that first come out from the mouth many, if asked whether access to such private members is possible, is: 'impossible!' or say the least: 'you can't do that!'. We are not talking about using accessors here, the usual getter/setter methods are completely excluded.
The truth about that is, gaining access to private members from an alien class is not only possible, but real.
May 15, 2012
How To Shutdown PC With Java Code
Subscribe to:
Posts (Atom)